PowerShell can be used as a REST client to access Azure REST APIs. To access Azure REST methods, you will need to have access to subscription with Azure AD App Registration. If you haven’t done Azure AD App registration. You can follow this article here. Make sure you capture client secret key after app is registered. Once you have tenant id
, client id
, client secret
, and subscription id
you can proceed forward with below instructions.
To make life easier, I have checked my PowerShell code on Github. Feel free to download them and modify them to your needs.
In this tutorial, I will go over how to get resource groups from Azure REST API. One of the basic CRUD operations, I will perform.
Create a file called Get-AzureResourceGroup.ps1
. Let’s create the required variables. Make sure to fill in the required variables from your Azure subscription.
Variables
# Variables
$TenantId = "" # Enter Tenant Id.
$ClientId = "" # Enter Client Id.
$ClientSecret = "" # Enter Client Secret.
$Resource = "https://management.core.windows.net/"
$SubscriptionId = "" # Enter Subscription Id.
Once you have updated the above required values. Let’s make a first REST call to get access token.
We will use the below URL to make a REST call to get access token.
POST https://login.microsoftonline.com/{tenantId}/oauth2/token
Request Access Token
Add the following code to your PowerShell script after variables.
$RequestAccessTokenUri = "https://login.microsoftonline.com/$TenantId/oauth2/token"
$body = "grant_type=client_credentials&client_id=$ClientId&client_secret=$ClientSecret&resource=$Resource"
$Token = Invoke-RestMethod -Method Post -Uri $RequestAccessTokenUri -Body $body -ContentType 'application/x-www-form-urlencoded'
Write-Host "Print Token" -ForegroundColor Green
Write-Output $Token
After you have retrieved the access token, we will use that to authorize Azure REST methods.
Get Resource groups
To retrieve all resource groups in Azure. We will use the below URL.
GET https://management.azure.com/subscriptions/{subscriptionId}/resourcegroups?api-version=2017-05-10
Enter below code to Get-AzureResourceGroup.ps1
.
# Get Azure Resource Groups
$ResourceGroupApiUri = "https://management.azure.com/subscriptions/$SubscriptionId/resourcegroups?api-version=2017-05-10"
$Headers = @{}
$Headers.Add("Authorization","$($Token.token_type) "+ " " + "$($Token.access_token)")
$ResourceGroups = Invoke-RestMethod -Method Get -Uri $ResourceGroupApiUri -Headers $Headers
Write-Host "Print Resource groups" -ForegroundColor Green
Write-Output $ResourceGroups
The above line of code should return all the resource groups from an Azure subscription.
Below is the full script and output.
# Variables
$TenantId = "" # Enter Tenant Id.
$ClientId = "" # Enter Client Id.
$ClientSecret = "" # Enter Client Secret.
$Resource = "https://management.core.windows.net/"
$SubscriptionId = "" # Enter Subscription Id.
$RequestAccessTokenUri = "https://login.microsoftonline.com/$TenantId/oauth2/token"
$body = "grant_type=client_credentials&client_id=$ClientId&client_secret=$ClientSecret&resource=$Resource"
$Token = Invoke-RestMethod -Method Post -Uri $RequestAccessTokenUri -Body $body -ContentType 'application/x-www-form-urlencoded'
Write-Host "Print Token" -ForegroundColor Green
Write-Output $Token
# Get Azure Resource Groups
$ResourceGroupApiUri = "https://management.azure.com/subscriptions/$SubscriptionId/resourcegroups?api-version=2017-05-10"
$Headers = @{}
$Headers.Add("Authorization","$($Token.token_type) "+ " " + "$($Token.access_token)")
$ResourceGroups = Invoke-RestMethod -Method Get -Uri $ResourceGroupApiUri -Headers $Headers
Write-Host "Print Resource groups" -ForegroundColor Green
Write-Output $ResourceGroups
Output:
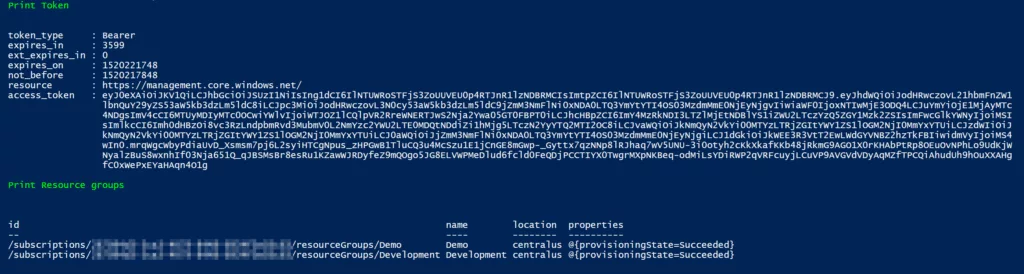